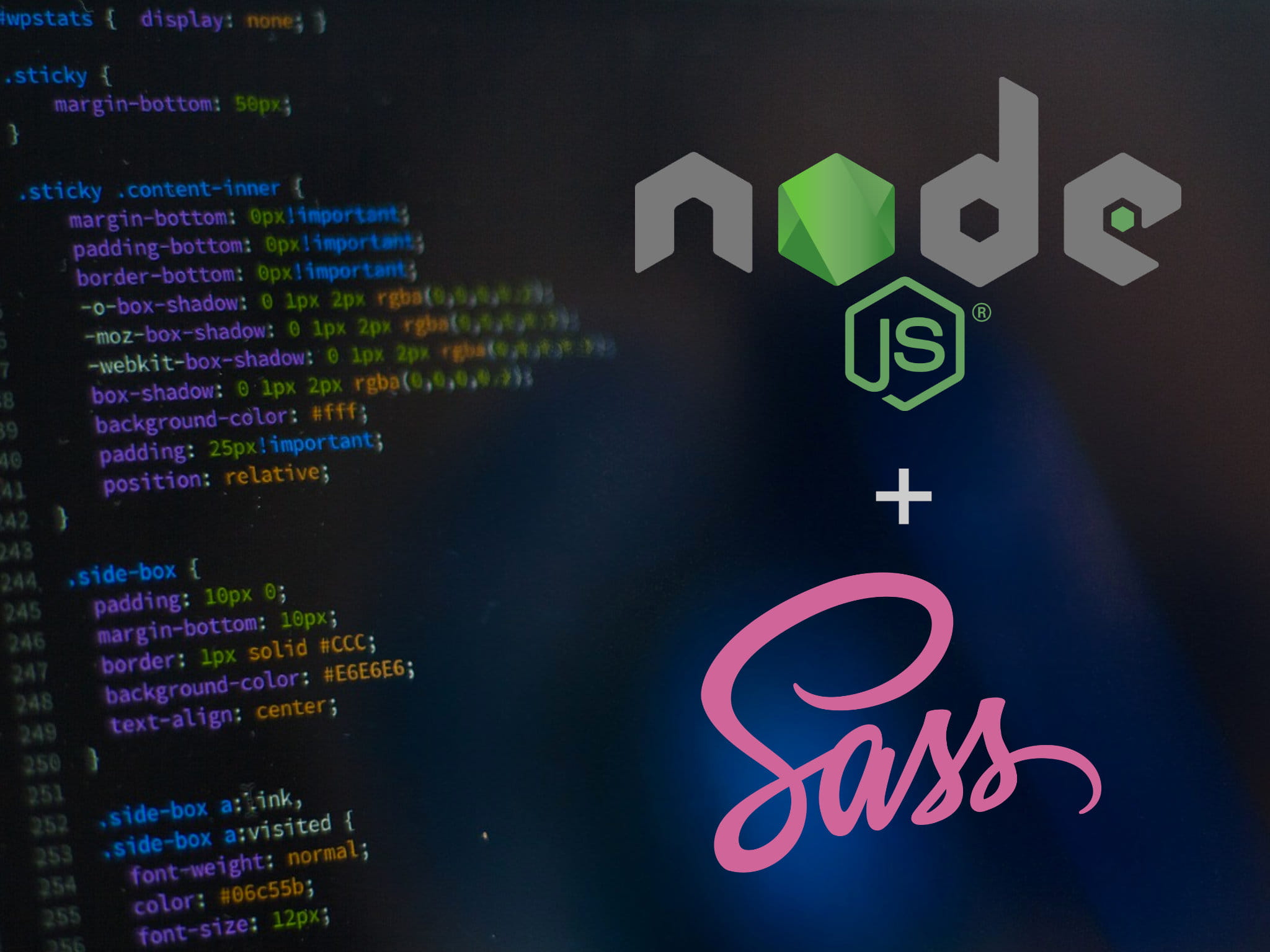
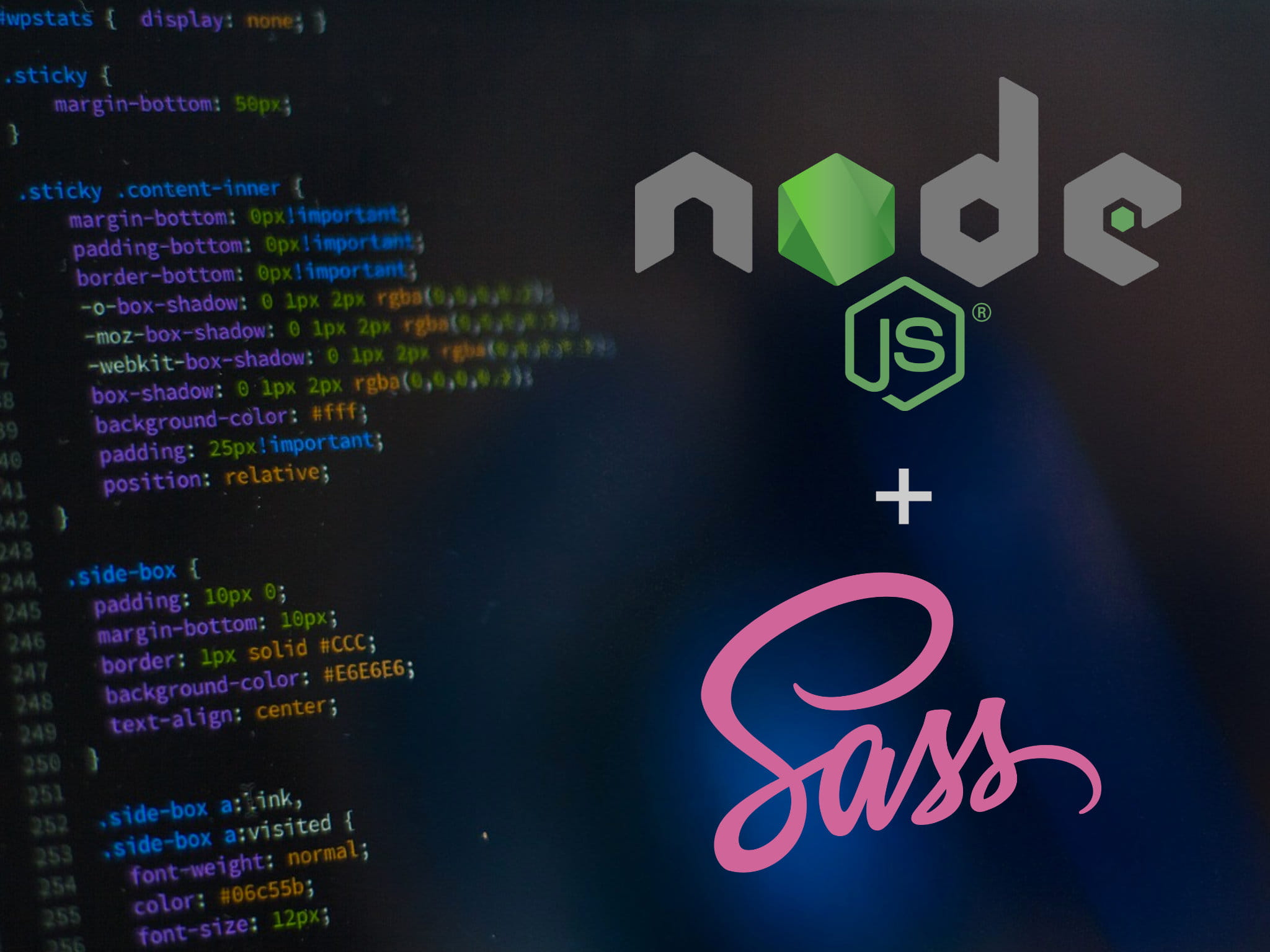
Sass is hands-down my favorite CSS pre-processor and it is commonly associated with writing a clean, reusable, and modular CSS. There are many SASS compiling tools available out there – paid as well as free to use. Personally, I have been using Koala for a long time as it is a great tool and it’s free.
Having said that, using Node.js and your Terminal to compile your SASS is definitely the most convenient way to do it.
Naturally, it can be a bit confusing meeting Node.js for designers who are just about to learn some coding, but it is quite easy to start. And once you get used to having such great power of npm packages under your fingertips, you will never look back.
To compile Sass via the command line, first, we need to install node.js. Download it from the official website nodejs.org, open the package, and follow the wizard.
NPM is the Node Package Manager for JavaScript. NPM makes it easy to install and uninstall third-party packages. To initialize a Sass project with NPM, open your terminal and CD (change directory) to your project folder.
Macintosh:~You$ cd Users/You/NewProject
Once in the correct folder, run the command:
npm init
You will be prompted to answer several questions about the project, after which NPM will generate a package.json file in your folder.
Node-sass is an NPM package that compiles Sass to CSS (which it does very quickly too). To install node-sass, run the following command in your terminal:
npm install node-sass
Now, everything is ready to write a small script in order to compile Sass. Open the package.json file in a code editor. You will see something like this:
{
"name": "sass-test-compiler",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo "Error: no test specified" && exit 1"
},
"author": "",
"license": "ISC"
}
In the scripts section add an SCSS command, below the test command, as it’s shown:
"scripts": {
"test": "echo "Error: no test specified" && exit 1",
"scss": "node-sass --watch scss -o css"
}
To execute this one-line script, we need to run the following command in the terminal:
npm run scss
And there it is. If you have followed all the steps successfully, watching and compiling SASS with Node.js should be up and running.